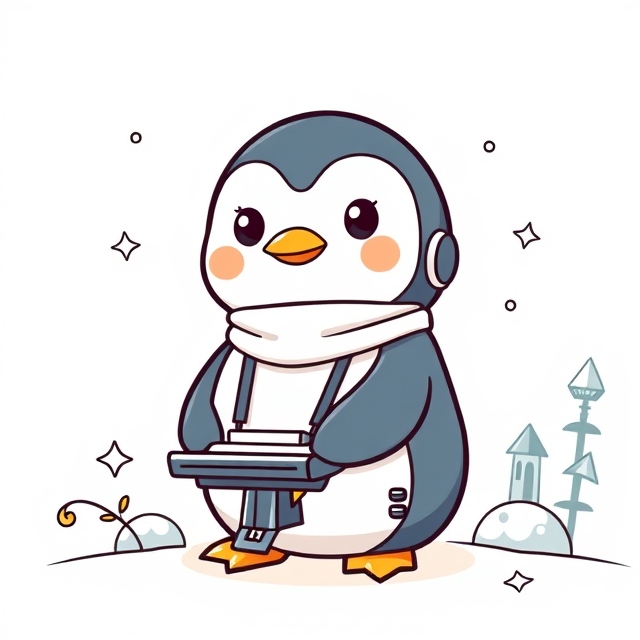
In-App Purchases and Subscriptions for Apple StoreKit 2 ✨
A lightweight, production-ready Swift package for validating StoreKit 2 in-app purchases and subscriptions. Built on top of Iaptic's enterprise-grade validation service.
Why Iaptic StoreKit 2?
🔒 Enterprise-Grade Security
- Real-time fraud detection
- Server-side validation
- Automated receipt refresh
💰 Subscription Management
- Real-time subscription tracking
- Automatic renewal handling
- Built-in grace period support
🚀 Smart Performance
- Intelligent caching system
- Automatic retry with backoff
- Thread-safe implementation
📱 Cross-Platform
- iOS, macOS, watchOS, tvOS
- Swift 5.5+ and StoreKit 2
- Modern async/await API
🔄 How It Works
sequenceDiagram
participant App
participant Iaptic
participant StoreKit
participant IapticServer
App->>StoreKit: Purchase product
StoreKit-->>App: Purchase result
App->>Iaptic: Validate purchase
Iaptic->>IapticServer: Send JWS representation
IapticServer-->>Iaptic: Validation result
Iaptic-->>App: Purchase validation details
App->>App: Grant entitlements
Note over App,IapticServer: App Launch / Refresh
StoreKit-->>App: Transaction updates
App->>Iaptic: Validate transactions
Iaptic->>IapticServer: Validate receipts
IapticServer-->>Iaptic: Validation result
Iaptic-->>App: Update entitlements
⚡️ Quick Implementation
// 1. Initialize the validator
import StoreKit
import Iaptic
let iaptic = Iaptic(
appName: "your-app-name",
publicKey: "your-public-key"
)
// 2. Validate purchases
let result = try await product.purchase()
switch result {
case .success(let verificationResult):
let validationResult = await iaptic.validate(
productId: product.id,
purchaseResult: result
)
if validationResult.isValid {
// Grant entitlements
}
}
// 3. Monitor subscription status
for await verificationResult in Transaction.updates {
if case .verified(let transaction) = verificationResult {
let validationResult = await iaptic.validate(
productId: transaction.productID,
verificationResult: verificationResult
)
if validationResult.isValid {
// Update entitlements
}
await transaction.finish()
}
}
🚀 Getting Started
Quick Links:
- API Documentation - Complete reference
- GitHub Repository - Source code and issues
- Demo Project - Implementation example
- Support - Get help when you need it
Requirements:
- iOS 15.0+, macOS 12.0+, watchOS 8.0+, tvOS 15.0+
- Swift 5.5+ with async/await support
- StoreKit 2
📦 Installation
Swift Package Manager
dependencies: [
.package(url: "https://github.com/iaptic/iaptic-storekit2.git", from: "1.0.0")
]